- Rsync
- Async - C# Reference | Microsoft Docs
- How To Use Rsync To Sync Local And Remote Directories ..
- Rsync Examples
- Rsync
Jerrick Leger is a CompTIA-certified IT Specialist with more than 10 years' experience in technical support and IT fields. He is also a systems administrator for an IT firm in Texas serving small businesses. ArRsync – an Rsync GUI for Mac OS X. Filed Under Computers & Tech on November 26, 2006 at 12:02 am. When it comes to efficiently synchronizing data between hard-drives there is a great Unix/Linux command-line tool, rsync, which is installed on OS X Tiger (and perhaps previous versions too) by default. OS X only contains the command-line tool.
-->Use the async
modifier to specify that a method, lambda expression, or anonymous method is asynchronous. If you use this modifier on a method or expression, it's referred to as an async method. The following example defines an async method named ExampleMethodAsync
:
If you're new to asynchronous programming or do not understand how an async method uses the await
operator to do potentially long-running work without blocking the caller's thread, read the introduction in Asynchronous programming with async and await. The following code is found inside an async method and calls the HttpClient.GetStringAsync method:
An async method runs synchronously until it reaches its first await
expression, at which point the method is suspended until the awaited task is complete. In the meantime, control returns to the caller of the method, as the example in the next section shows.
If the method that the async
keyword modifies doesn't contain an await
expression or statement, the method executes synchronously. A compiler warning alerts you to any async methods that don't contain await
statements, because that situation might indicate an error. See Compiler Warning (level 1) CS4014.
The async
keyword is contextual in that it's a keyword only when it modifies a method, a lambda expression, or an anonymous method. In all other contexts, it's interpreted as an identifier.
Example
The following example shows the structure and flow of control between an async event handler, StartButton_Click
, and an async method, ExampleMethodAsync
. The result from the async method is the number of characters of a web page. The code is suitable for a Windows Presentation Foundation (WPF) app or Windows Store app that you create in Visual Studio; see the code comments for setting up the app.
You can run this code in Visual Studio as a Windows Presentation Foundation (WPF) app or a Windows Store app. You need a Button control named StartButton
and a Textbox control named ResultsTextBox
. Remember to set the names and handler so that you have something like this:
To run the code as a WPF app:
- Paste this code into the
MainWindow
class in MainWindow.xaml.cs. - Add a reference to System.Net.Http.
- Add a
using
directive for System.Net.Http.
To run the code as a Windows Store app:
- Paste this code into the
MainPage
class in MainPage.xaml.cs. - Add using directives for System.Net.Http and System.Threading.Tasks.
Important
For more information about tasks and the code that executes while waiting for a task, see Asynchronous programming with async and await. For a full console example that uses similar elements, see Process asynchronous tasks as they complete (C#).
Return Types
An async method can have the following return types:
- void.
async void
methods are generally discouraged for code other than event handlers because callers cannotawait
those methods and must implement a different mechanism to report successful completion or error conditions. - Starting with C# 7.0, any type that has an accessible
GetAwaiter
method. TheSystem.Threading.Tasks.ValueTask<TResult>
type is one such implementation. It is available by adding the NuGet packageSystem.Threading.Tasks.Extensions
.
The async method can't declare any in, ref or out parameters, nor can it have a reference return value, but it can call methods that have such parameters.
You specify Task<TResult>
as the return type of an async method if the return statement of the method specifies an operand of type TResult
. You use Task
if no meaningful value is returned when the method is completed. That is, a call to the method returns a Task
, but when the Task
is completed, any await
expression that's awaiting the Task
evaluates to void
.
You use the void
return type primarily to define event handlers, which require that return type. The caller of a void
-returning async method can't await it and can't catch exceptions that the method throws.
Starting with C# 7.0, you return another type, typically a value type, that has a GetAwaiter
method to minimize memory allocations in performance-critical sections of code.
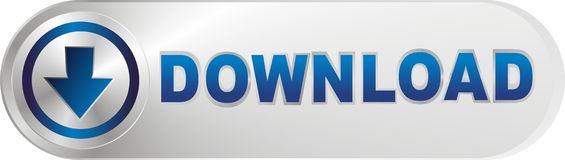
For more information and examples, see Async Return Types.
See also
Tutorial
Introduction
Rsync, which stands for “remote sync”, is a remote and local file synchronization tool. It uses an algorithm that minimizes the amount of data copied by only moving the portions of files that have changed. Genymotion android emulator for mac.
In this guide, we will cover the basic usage of this powerful utility.
What Is Rsync?
Rsync is a very flexible network-enabled syncing tool. Due to its ubiquity on Linux and Unix-like systems and its popularity as a tool for system scripts, it is included on most Linux distributions by default.
Rsync
Basic Syntax
The basic syntax of rsync
is very straightforward, and operates in a way that is similar to ssh, scp, and cp.
We will create two test directories and some test files with the following commands:
We now have a directory called dir1
with 100 empty files in it.
We also have an empty directory called dir2
.
To sync the contents of dir1
to dir2
on the same system, type:
Async - C# Reference | Microsoft Docs
The -r
option means recursive, which is necessary for directory syncing.
We could also use the -a
flag instead:
The -a
option is a combination flag. It stands for “archive” and syncs recursively and preserves symbolic links, special and device files, modification times, group, owner, and permissions. It is more commonly used than -r
and is usually what you want to use.
An Important Note
You may have noticed that there is a trailing slash (/
) at the end of the first argument in the above commands:
This is necessary to mean “the contents of dir1
”. The alternative, without the trailing slash, would place dir1
, including the directory, within dir2
. This would create a hierarchy that looks like:
Always double-check your arguments before executing an rsync command. Rsync provides a method for doing this by passing the -n
or --dry-run
options. The -v
flag (for verbose) is also necessary to get the appropriate output:
Compare this output to the output we get when we remove the trailing slash:
You can see here that the directory itself is transferred.
How To Use Rsync to Sync with a Remote System
Syncing to a remote system is trivial if you have SSH access to the remote machine and rsync
installed on both sides. Once you have SSH access verified between the two machines, you can sync the dir1
folder from earlier to a remote computer by using this syntax (note that we want to transfer the actual directory in this case, so we omit the trailing slash): Bluestacks download for macbook air.
This is called a “push” operation because it pushes a directory from the local system to a remote system. The opposite operation is “pull”. It is used to sync a remote directory to the local system. If the dir1
were on the remote system instead of our local system, the syntax would be:
Bluestack m1 chip. Like cp
and similar tools, the source is always the first argument, and the destination is always the second.
Useful Options for Rsync
Rsync provides many options for altering the default behavior of the utility. We have already discussed some of the more necessary flags.
If you are transferring files that have not already been compressed, like text files, you can reduce the network transfer by adding compression with the -z
option:
The -P
flag is very helpful. It combines the flags --progress
and --partial
. The first of these gives you a progress bar for the transfers and the second allows you to resume interrupted transfers:
How To Use Rsync To Sync Local And Remote Directories ..
If we run the command again, we will get a shorter output, because no changes have been made. This illustrates rsync’s ability to use modification times to determine if changes have been made.
We can update the modification time on some of the files and see that rsync intelligently re-copies only the changed files:
In order to keep two directories truly in sync, it is necessary to delete files from the destination directory if they are removed from the source. By default, rsync does not delete anything from the destination directory.
We can change this behavior with the --delete
option. Before using this option, use the --dry-run
option and do testing to prevent data loss:
If you wish to exclude certain files or directories located inside a directory you are syncing, you can do so by specifying them in a comma-separated list following the --exclude=
option:

If we have specified a pattern to exclude, we can override that exclusion for files that match a different pattern by using the --include=
option.
Finally, rsync’s --backup
option can be used to store backups of important files. It is used in conjunction with the --backup-dir
option, which specifies the directory where the backup files should be stored.
Conclusion
Rsync Examples
Rsync can simplify file transfers over networked connections and add robustness to local directory syncing. The flexibility of rsync makes it a good option for many different file-level operations.
Rsync
A mastery of rsync allows you to design complex backup operations and obtain fine-grained control over what is transferred and how.